Intro to Computing (ECE120)
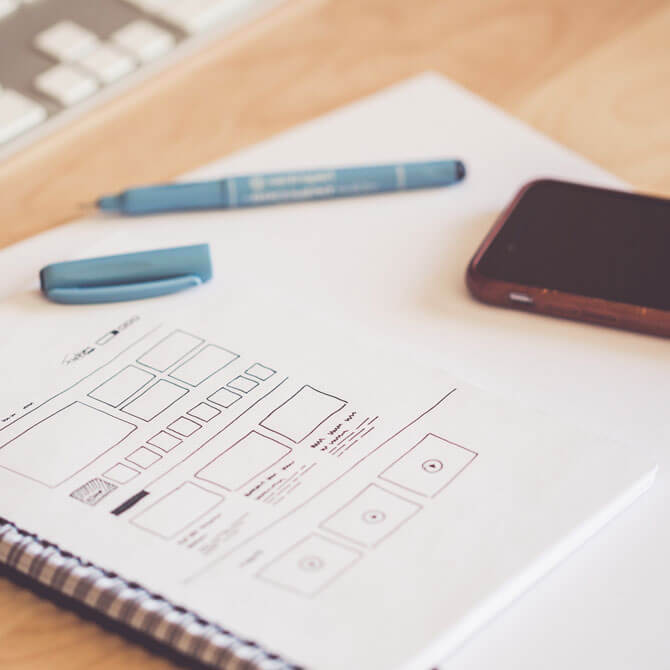
About this course
ECE 120 - Introduction to Computing
Introduction to digital logic, computer systems, and computer languages. Topics include representation of information, combinational and sequential logic analysis and design, finite state machines, the von Neumann model, basic computer organization, and machine language programming. Laboratory assignments provide hands-on experience with design, simulation, implementation, and programming of digital systems. Course Information: Prerequisite: Restricted to Computer Engineering or Electrical Engineering majors or transfer students with ECE Department consent.
Detailed Description and Outline
ECE 120 gives an introduction to the design and programming of computing systems. We start the course by motivating our objectives and connecting them with students’ future ECE studies and career paths. The philosophy of our approach is quite different than the typical introduction to programming course: after a brief illustration of our goals and objectives with a quick introduction to C, we approach programming from the bottom upwards. In particular, we begin by describing the architecture of a computer, including logic gates, datapaths, registers, and memory. Throughout the course, we will make connections between hardware and software and explore the engineering tradeoffs in using each to develop computing systems.
Outline:
- Abstraction, bits, unsigned representation, signed integers, 2’s complement representation, fixed- and floating-point representation, hexadecimal notation, ASCII representation, unsigned binary addition, modular arithmetic, carry out, overflow
- Introduction to digital logic, CMOS, logic gates, truth tables, Boolean logic operations, Karnaugh maps, sum-of-product, product-of-sums, logical completeness, Boolean properties, two-level design, Pareto optimization, don’t care simplification
- Introduction to UNIX, introduction to C programming (operators, functions, statements), flow chart, sequential construct, conditional construct, iterative construct, program execution, program analysis, program testing
- Bit-sliced design, ripple-carry adder, bit-sliced comparator, 2’s complement comparator, building with abstraction, multiplexers, decoders
- Clock abstraction, latches, flip-flops, shift registers, registers with parallel load, serialization
- Finite state machines (FSM), binary counters, FSM models, clock synchronous design, modular FSM design (keyless car entry, vending machine)
- Concept of memory, address space, addressability, building larger memory using smaller memory, coincident selection, tri-state buffer
- The von Neumann model, LC-3 as von Neumann, instruction processing, instruction set architecture, control unit design, assemblers and assembly code
- Error detection and correction, odd/even parity bit, Hamming distance, Hamming code
Homework
Introduction to C programming
- C programming: Basic I/O, control structures in C
- C programming: Program execution, program analysis, program testing
Boolean algebra and two-level design
- Boolean properties; don’t care simplification
- Combinational logic structures: ripple-carry adder; bit-sliced design
Design of combinational logic circuits
- Combinational logic structures: bit-sliced comparator
- Abstraction in design
- Combinational logic structures: decoders, muxes
Sequential logic elements
- Storing a bit: latches and flip-flops
- Static Hazards; shift registers, registers with parallel load
- Serialization
Sequential logic, synchronous design
- Finite state machines; binary counters
- FSM models; Clock synchronous design
Component-based FSM Design; Memory
- Concept of memory
- From FSM to computer
- The von Neumann model: basic components, instruction cycle
The Von Neumann Model; LC-3 ISA and programming in binary machine language
- Instruction formats and instruction processing
- LC-3 ISA
- LC-3 binary program analysis: counting to 10
LC-3 Programming in binary machine language
- Programming in LC-3 machine language: typing a number
- Problem solving using systematic decomposition; Good design
- Programming example: letter frequency counter
LC-3 programming in assembly
- Introduction to LC-3 assembly language; the assembly process
- LC-3 assembly programming: ASCII screen art example
LC-3 datapath and control word
- Design of the LC-3 datapath; fetch and decode execution
- LDI control signals example; hardwired control unit design
- Microprogrammed control unit design; the Patt and Patel control unit